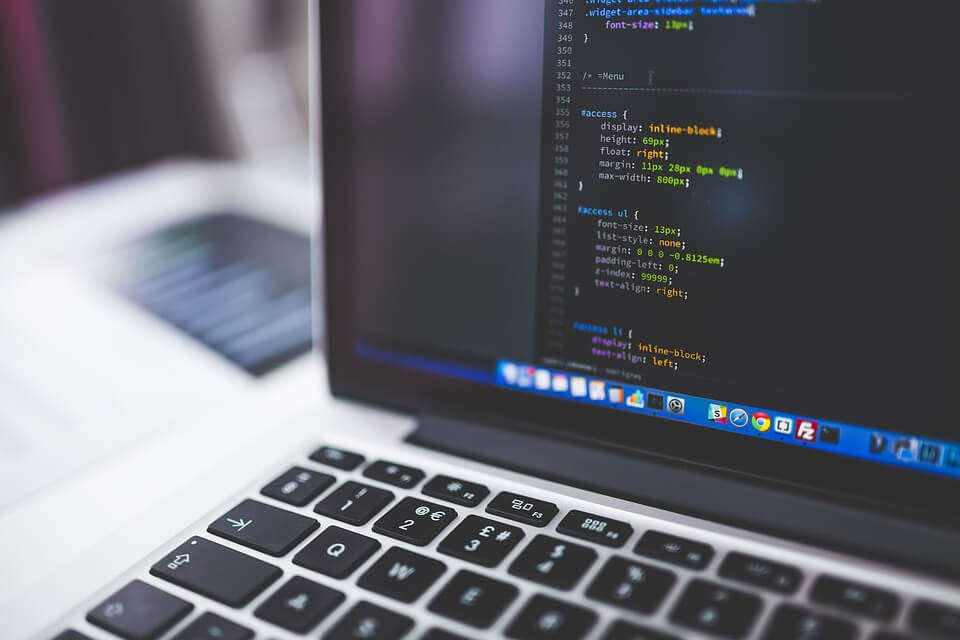
Typical back-end architecture for mobile app
Certainly, software project development is a complicated process consisting of various stages. It also has a typical architecture, including backend and frontend parts and requiring a wide range of tools and technologies to be built.
It’s important to be aware of how a software project architecture looks like: you may need this knowledge when preparing a technical specification and deciding on what technology stack to use. You will also get an understanding of what specialists you would need for your future project.
In this post, we’ll describe a typical product architecture. What’s more, we’ll share the principles that describe our app development process as well as proven tools that we use to deliver quality solutions. So, let’s start.
1. Backend
1.1 Server infrastructure
We use a wide range of services provided by Amazon Web Services (AWS) for our server infrastructure. AWS has established itself as a quite reliable service provider. It can be used in a variety of projects, allowing to create secure and scalable solutions.
We use Terraform tool for infrastructure configuration, enabling our app development team to describe it as a code and reuse this configuration few times for the creation of some new infrastructures.
Each project generally has 2 application infrastructures:
- Development (staging)
- Production (live)
Each configuration has VPC (Virtual Private Cloud) with sub networks and route tables to establish security and resources isolation.
In our projects we use the following services and abilities provided by Amazon Web Services:
- Amazon VPC
- Amazon EC2
- Amazon ECS
- Amazon Application Load Balancer
- Amazon Auto Scaling
- Amazon Lambda
- Amazon RDS
- Amazon ElastiCache
- Amazon S3
- Amazon SQS
- Amazon SNS
- Amazon SES
- Amazon Route 53
- Amazon Certificate Manager
- Amazon CloudFront
Amazon VPC is a service that provides the ability to create a virtual private cloud in Amazon and isolate all resources. By using this service we ensure the security of our resources in Amazon cloud.
Amazon EC2 is a service providing server instances for different purposes. We use server instances with Ubuntu operation system or Amazon Linux optimized for using by Amazon ECS.
Amazon ECS provides Docker container orchestration possibilities in Amazon infrastructure. It represents an Amazon analog for Kubernetes orchestration tool.
Amazon Application Load Balancer allows to automatically distribute incoming app traffic across multiple Amazon application instances deployed to ECS cluster or EC2 instances.
Amazon Auto Scaling is a service that helps maintain app availability and allows scaling EC2 instances (with an application or in ECS cluster) capacity up or down automatically according to the determined conditions (CPU or Network traffic).
Amazon Lambda enables a serverless approach in Amazon infrastructure. We use this service to execute some short live tasks or cron actions that can work independently on the main application to prevent these actions from consuming the principal server resources. In our work, we don’t need any server configuration on instances to execute these tasks.
Amazon RDS includes a database for the application. Generally, we use a Postgres database, as it demonstrates good performance and provides developers with a wide range of opportunities.
Amazon ElastiCache provides already configured and production ready In Memory Database such as Redis or Memcache. We use this service to store our application caches.
Amazon S3 is used for all kind of files.
Amazon SQS is a message queue service that provides functionality similar to JMS. Thanks to Amazon SQS we get the ability to decouple app components.
Amazon SNS is primarily used for sending push notification on different platforms.
Amazon SES service is used for email sending.
Amazon Route 53 represents a cloud DNS service that effectively connects user requests to the infrastructure, running in AWS – such as Amazon EC2 instances, Application Load Balancers, Amazon S3 buckets or Amazon CloudFront distributions.
Amazon Certificate Manager enables to store any certificates and connect them to Amazon CloudFront or Amazon Application Load Balancer. With ACM, you can upload the already existing certificate to this service or use certificates that are freely generated by Amazon.
Amazon CloudFront is a service that provides CDN functionality in Amazon. We can connect this service with Amazon S3 and distribute files with caching in some edge locations provided by Amazon. This service also enables developers to automate files distribution.
Common application infrastructure illustrated in the diagram below:
1.2 Backend application architecture
Application usually has a monolith architecture with layers for code decoupling.
We have the following layers:
- Repository layer. This layer has classes that implement database communication functionality such as requests or updates through database models.
- Services layer. It has classes that implement application business logic, integration with 3rd party systems, transactions handling, and caching.
- Controller layer. This layer has classes that implement HTTP requests handling. HTTP API is implemented according to RESTful principles and can work with different request content types such as JSON, XML, and protobuf.
1.3 Backend technology stack
We develop applications for a JVM platform with Java 8 programming language. We use cutting-edge technology stack that includes the following libraries/frameworks/tools:
- PostgreSQL
- Spring Boot
- Gradle
- Flyway
- JOOQ
- Lombok
- Javaslang
- HikariCP
- Spring Rest Docs/Swagger
- Jackson
- SLF4J with Logback
- Docker
PostgreSQL is a modern database that provides high performance, follows SQL standard and has a lot of useful features such as support for geospatial data with PostGIS extension and json data type.
Spring Boot is a core framework in our applications. This framework provides a wide range of abilities: setup Inversion Of Control support, the implementation of many integrations with 3rd party systems, the ability to build HTTP controllers and handle transactions.
Gradle is a build tool we use in our projects. It has flexible DSL allowing us to configure project build process and integrate it with some external libraries or systems. We use many Gradle plugins to set up our programming flow.
Flyway is a database version control system that enables us to define all SQL DDL or other statements in its evolution files.
JOOQ is a database access framework that works on repository layer and generates Java model classes from database schema for communication with a database.
Lombok is a library that helps remove some boilerplate code and generate this code for runtime by Java agent.
Javaslang is a functional library for Java. This tool helps us reduce the amount of code by using a functional approach in some cases.
HikariCP is a modern database connection pool that holds opened database connections and reduces database queries execution time by removing the necessity to open a new connection each time. This connection pool has good performance and flexible configuration comparing to other connections pools.
Spring REST docs/Swagger is used for automatic REST API documentation generation.
Jackson is a library for objects serialization/deserialization.
SLF4J with Logback (SLF4J) serves as a simple facade/abstraction for different logging frameworks like java.util.logging, logback and log4j.
Docker is a technology that ensures our backend delivery model through containers. Our backend application has built to a self-executable jar file and wrapped to Docker images with all required configurations for containers. These docker images with an application can be easily run on any infrastructure or environment without any pre-setup on instance configuration.
1.4 Backend development process
There are some principles that describe our app development process:
- We use git as VCS and follow git flow in our software development process.
- Each project has .editorconfig file to define some basic consistent coding styles between different editors and IDEs for all project developers.
- We have integration of flyway database version control tool and jooq code generation in software development process. If we have some changes in a database schema during the development then just after automatic build we have applied new SQL evolution in a database and out to date database model classes in project source code.
- All new business functionality is covered by unit test with mocks. We use a Junit framework for this tests, Mockito for mocks and AssertJ for data assertions.
- We also have integration tests for some REST API controllers that check all system integrity. Spring REST Docs tool allows our app development team to create integration tests and generates API document according to test results. Test execution is attached to build process.
- We use jacoco to generate tests code coverage report. Report generation is attached to build process.
- Code quality is checked with SonarQube software. Project build system has integration with SonarQube plugin and sends code quality information to a remote SonarQube instance. In accordance with Sona Qube report, we can ensure that our project code doesn’t have any ‘code smell’, ‘technical debts ’ or security problems. SonarQube also shows analyze jacoco reports and provides all information in one place.
- We also use CI/CD practices in app development process and use Jenkins with different plugins that help us to organize jobs for this purpose. Jenkins is configured to run application build after commits to git automatically and also has some jobs for release purposes.
- Our app development team applies Prometheus to gather all servers metrics and generate alerts and Grafana to visualize metrics data. This tool helps us quickly define possible problems in the system.
- We use ELK stack to gather, store and visualize application logs. We can find detailed information about problems in our system with this tool set.
2. Clients
We usually have several types of clients: mobile (iOS/Android) and web. All clients communicate with backend through RESTful web services. API has self-generated documentation provided by Spring Rest Docs or Swagger.
Spring Rest Docs
Swagger
2.1 Web client
Usually, we have separated from the main application web client that developed as a static single page app that has javascript, CSS, HTML code that runs on web browser side.
In our app development process, we decided to avoid using web servers such as Nginx or Apache and use Amazon S3 and all its capabilities to store and expose to internet this application.
As a result, we have unlimited throughput and low latency with minimal configuration and without any servers for frontend application.
As the main framework for a web client, we use an angular framework.
Application dependencies are provided by bower package manager.
During app development process we have source code obfuscation phase.
2.2 Mobile client
We build mobile applications for both iOS and Android, that now are the most popular operating systems. Noteworthy that they also use backend REST API.
In iOS development, we primarily use Swift programming language, for its effective features, convenience, and speed (learn, how to successfully migrate your project to Swift 4).
What concerns the other platform, we build Android apps on Java and Kotlin, since they both enable to deliver quality, reliable, and scalable solutions.
Specializing in custom mobile and web app development, we are ready to provide you with a free consultation to your project. Feel free to apply to us!